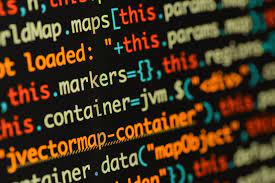
The first steps can be intimidating for many developers who have never built a JavaScript web application before. Our development team has the opportunity to work with both new students and seasoned developers who have extensive experience developing complex applications.
Even seasoned back-end developers frequently inquire about where they can learn JavaScript. Invariably, our response is, “Don’t limit yourself to reading. You must begin building things and experimenting with the language to see what it is capable of.”
JavaScript Frameworks
They frequently inquire, “Which framework should I learn?” Angular, Vue, and React are popular JavaScript frameworks, but they can make it difficult to know where to begin. Many developers may not want to choose a framework at this point in order to avoid being locked into a specific technology.
You’re not alone if you’re curious about these topics. Fortunately, there are numerous free resources available to assist you in learning how to create enterprise-grade JavaScript applications.
The other good news is that the JavaScript framework you choose should have no effect on the user experience of your application in the end. When you prioritize your content and information architecture, the framework becomes a minor consideration.
From personal experience, it’s easy to get excited about a specific framework, but in a fast-changing environment, this can lead to long-term disappointment. Instead, learning the fundamentals of JavaScript will set you up for successful web development in the future.
With that in mind, I’d like to outline a strategy for preparing for a career in front-end development. Many of the topics I’ll cover are applicable across the entire JavaScript ecosystem, and the skills you’ll learn here are transferable. Instead of just explaining how to get started with these, I wanted to compile a list of resources that could be helpful.
The application developer development process, in my opinion, has two basic steps. The JavaScript ecosystem is learned first, followed by web application architecture. Learning JavaScript and practicing JavaScript coding are both important parts of understanding the JavaScript ecosystem. After that, you can start working on your first Node.js application.
Understanding web application architecture, the second step, also has two stages. You’ll need to apply your JavaScript skills to creating a web application and making code architecture decisions. Then you can decide how to build and deploy your application. Let’s take each of these steps one at a time. I won’t go into too much detail; instead, I’ll outline the steps and point you to resources that can assist you in making these decisions.
JavaScript Ecosystem
We’ll begin with the first two stages of the first step, which will lead to the creation of your first Node.js application.
Develop an understanding of JavaScript and practice writing JavaScript code
The Mozilla Developer Network (MDN) is an excellent resource for learning JavaScript. This 30- to 60-minute JavaScript reintroduction can help with a high-level overview of the fundamentals. It’s critical to go deeper into the language once you’ve mastered the fundamentals. This will take some time, but knowing the power of JavaScript and some of the language’s quirks will be invaluable.
Understanding JavaScript also serves as a solid foundation for any front-end development project. In order to achieve interactivity, all frameworks that target browsers end up using JavaScript in some form or another. The MDN documentation has a much more in-depth tutorial if you want to go deeper.
You Don’t Know JS Yet has also proven to be an invaluable resource for developers looking to expand their JavaScript knowledge. This resource is described as “a series of books delving deep into the core mechanisms of the JavaScript language” by the author.
Starting out with a Node application
It’s likely that the tutorials mentioned above resulted in a Node application to run your JavaScript now that you’ve mastered the language. If you don’t know what Node.js is, it’s a good idea to learn about it. Although browsers can run JavaScript without assistance, a web application that relies on open source libraries and makes use of asset compilation and bundling is required. This requirement necessitates the use of Node.js. To get started with Node.js, I recommend starting with this introduction to the language. Then there’s information on how to install Node.js locally and get started on Nodejs.dev.
JavaScript files can be executed outside of the browser using Node. It’s also an important part of creating and using web applications. You may be familiar with NPM, a package manager included with Node. The package.json file, which is also described in detail on the Nodejs.dev site, is an important part of NPM.
Most developers will become very familiar with running npm init in an empty directory. This action is almost always the first step in building a Node-based web application. Once the package.json file exists, you can add commands to the scripts: {} section to execute commands. For example, you could add something like this:
"scripts": { "hello": "echo "hello"" }
Save the package.json file. Then, from the command line, run:
$ npm run hello
You should see the output: “hello”. The scripts section of this file is powerful. I encourage you to become familiar with package.json and how to execute commands using it.
It’s time to start building your JavaScript web application now that you know the basics of JavaScript and how to use Node.js at a high level. There are numerous options available, but let’s move on to the next steps without going too far down this rabbit hole.
Understanding web application architecture
Let’s move on to the second step’s two stages, which lead to your application’s build and deployment decisions.
Transition JavaScript skills to web applications and their architecture
Considering your application architecture before writing a single line of code is a good place to start. Many applications have had to be refactored because the developer failed to consider how their application would scale. When there are only five files in a component folder, it appears organized, but once there are 100 files, it’s difficult to find what you’re looking for. Any decision that has the potential to affect every single JavaScript file you create should be carefully considered in advance.
It’s easy to become overwhelmed by this process and become stuck in a never-ending cycle of indecision. You can break free by identifying existing tooling and learning from the experiences of others who have done this before you. To that end, because you’ll be making a lot of decisions while developing your software, here are some things to think about before you start writing any code:
- JavaScript framework choice
- File/folder structure for expansion
- CSS architecture
- Typescript or not
- Code linting
- Test approach
- Routing
- State management and caching layer
Make build and deployment decisions for your application
After you’ve decided on your basic code architecture, you’ll need to decide how developers will build and work with the code. You’ll also need to consider how the final code will be compiled for delivery to production. These subsequent decisions may be made easier if earlier framework choices have been made: There are frequently built-in tools that come with those frameworks.
I’ve also discovered that because these layers are generally outside of the code, they’re easier to change later. When considering the following, using standard tooling options like webpack or gulp can help a lot:
- Local developer environment
- Source maps
- Module bundler
- Production optimization
Final Thought
Working with the JavaScript ecosystem is a lot of fun, but it evolves quickly. While many of us prefer stability, focusing on the fundamentals and then applying that knowledge to your web applications will help you become a successful web application developer.